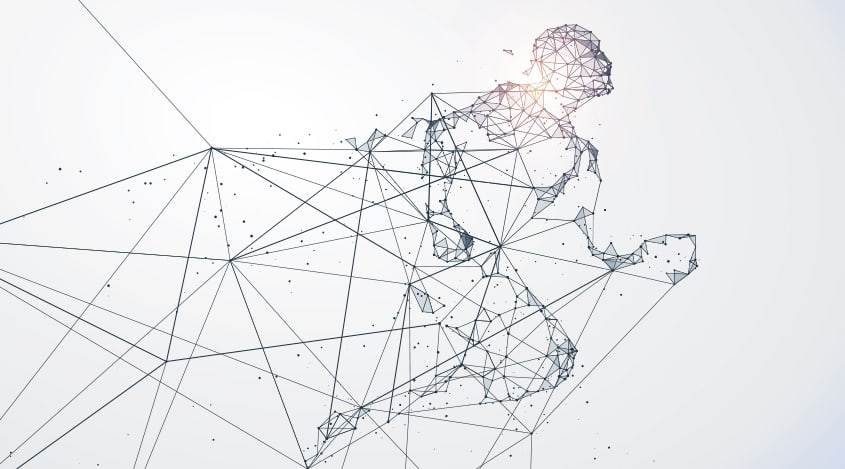
Basics of the MQL4 language for beginners
Friday, 5 January 2018 10:38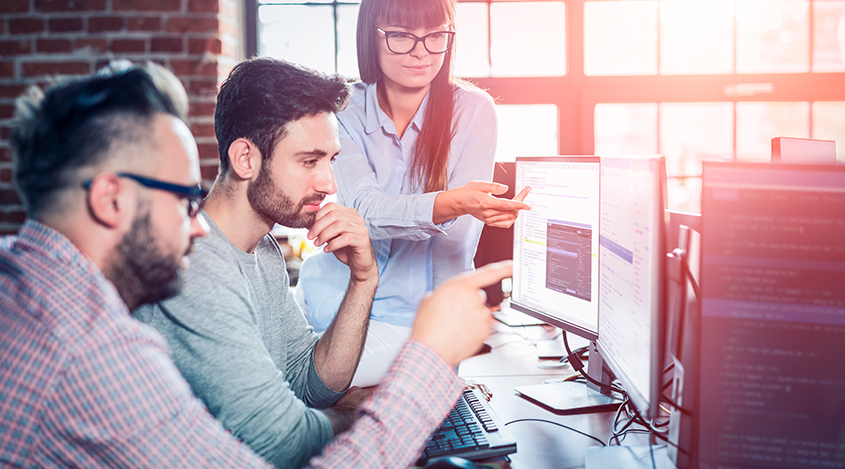
Over the lifetime of the MetaTrader terminal, a huge number of scripts, advisors and indicators have been created. They are freely available on various resources, including MT4 and MT5 trading platforms. Anyone can use them. Nevertheless, sooner or later each trader thinks about creating his own trading robot.
If you have at least a minimal knowledge of programming, you will quickly master the MQL4 language and start writing your own tools. But, if you have no idea, how the codes are written and you want to understand the basics of the MQL4 language, this article will be extremely useful for you.
What can I do using MQL4?
The MQL4 programming language allows you to create the following types of tools:
- Scripts are programs that perform a one-time mechanical action. They can open opposite orders, close trading positions, display information on the chart, etc. Scripts facilitate the work of a trader, making routine work for him.
- Custom indicators are technical analysis tools that are displayed on the terminal screen in the form of a graph or a histogram with each price change (tick). Unlike standard programs built into MetaTrader 4, custom indicators reflect exactly information that you need. They allow you to get rid of unnecessary indicators.
- Trade advisers are more complex programs. They simultaneously combine the properties of scripts and indicators. An adviser analyzes the market situation and performs actions in accordance with the algorithm embedded in its code. Writing your own robot, you can use personal experience, if you think that other people's strategies are not so effective as yours.
- Library is a list of functions that your trading robot will use when certain tasks perform. It can be standard mathematical function or your authoring development, according to which your adviser will open and close positions.
As you can see, knowledge of the MQL4 language gives the trader additional advantages. You will be able to create tools based on your own trading strategy. This is much more convenient than adjusting it to other people's designs.
How to create a trading robot
Scripts, indicators and other trading robots are created in MetaTrader 4 application - MetaEditor 4. To call it, you need to press F4 when the client terminal is open. The designer window will appear:
To create the tool, go to the "File - New" menu or press the keyboard shortcut Ctrl + N.
After that, select the desired tool and click the "Next" button.
In the appeared window, you should assign the name of the script (indicator, advisor), the author's name, the website address in the Link line. Then, click the "Finish" button.
Next, a window for creating a trading robot will appear. Now, you can proceed directly to writing the code. In the next section, we'll look at its main elements.
Written code will be clear to you, but the MetaTrader terminal doesn't understand it. To translate the source code, you wrote to the MT4 understandable language, you need to compile it. To do this, press F5 while the MetaEditor window is opened. After that, double-click on the trading robot's name in the MetaTrader menu and check its functionality.
Programming of trading robots: basic concepts
The code of the trading robot, like the code of any other program, is a specific syntax that contains the following elements:
- variables;
- comments;
- functions;
- arrays;
- cycles;
Let's consider each of them in more detail.
Variables
From the mathematics lessons you probably remember that a variable is an indicator that can take any of a number of values. In programming, this term means an area in the computer's memory in which data of a certain type is stored. This concept seems to be quite complex in theory. So, let's consider an example.
The age of any person changes over time. So, age is a variable according to the mathematical point of view. When writing a code, a variable named "Age" will store data about how the value of this parameter has changed over time.
Age is usually denoted by an integer. So, it will refer to the type of variables "int". If you display the variable "Age" using the MQL4 language, you'll get: "int age = 20".
But, if we work with parameters whose value is displayed in fractional numbers, (for example, height) we need another type of variables - "double". Example: "double height = 1.65".
There are also other variables:
- "string" is a data type whose values are a string consisting of alphabet characters (Example: string name = "Bob"). Please note, alphabetic values are written in quotation marks;
- "bool" is a type of variables that can take only one of two values: true or false. Example: bool trend = true.
In MQL4, variable names are often denoted by the letters "a", "b", "c", etc.
Important: When writing the code in MQL4, it is necessary to keep the register: the same variable names written in lowercase and uppercase letters will be perceived by the system as two different variables. The variable name cannot begin with numbers or special characters. Otherwise, the program will not be able to recognize them.
Comments
Comments are one of the important elements of the MQL4 code. They are not perceived by the program, but they are useful to the trader. He can obtain information about the variable using a comment. To denote this element, you need to put "//" before it. Thus, a comment will not be accepted by MT4 after compilation, but it will remain as a hint in the code. Example:
Functions
A function is a code snippet that returns predefined parameters from anywhere in the program. When writing scripts in MQL4, the Message Box function is often used. The function displays a message on the screen. It can contain information about the value of variables or output the result of calculations on the given parameters. For example, if in the code we specify:
After compiling the program, we get:
Functions are often used in all programming languages because they allow to reduce the amount of source code.
Arrays
An array is another element that allows you to reduce the amount of source code. It combines several identical variables that follow one another and have corresponding indices (0, 1, 2, 3, etc.). For example, we need to remember five prices:
Here we see several values of the same parameter - the price. We can combine them into one array: "double price [5]" - an array of five elements. In the future, it will be enough to prescribe only an element that you need to address in this section of the program.
The array also allows you to reduce the amount of code, if you declare each element an initial value: double price [2] = {1.3525, 1.3548}. In this case, it is not even necessary to specify the number of elements "2". The compiler will set the desired value independently.
Cycles
A cycle is a code element that triggers a large number of operations until the conditions for stopping them are reached. For example, we need to calculate the arithmetic average of the maximum price of all bars on the chart. In addition, the program must to repeat this action until the average price is greater than the value of "Bars". Without using a cycle, you will have to insert a huge number of variables every time:
But, if you convert this data into a cycle and specify the conditions under which it will be executed, the code volume will be significantly reduced:
The cycle begins with the word "for". It means that in this case the increment of the counter (int a = 0) will be executed by one step every time. There are also more complex types of cycles - while and repeat. They allow you to assign the counter individual conditions.
Conditions
The "if" element allows you to program the trading robot to do an action when certain conditions occur. It is often used with the "else" command. For example:
In the code above, it is indicated that b = 1 only when a> 10. Otherwise, b = 2. Since the given value a = 10 (not more than 10), b will conform to "else" or 2.
The conditions help to set the algorithm of the adviser's work: the robot will open positions only when the price corresponds to the specified conditions.
When writing trading robots, there are often situations when you need to immediately set several conditions for opening a deal. In MQL4, you can implement this by specifying every next parameter after "&&". Example: "if ((a> 5) && (b <10))".
So, we have considered the main elements of MQL4 programming language. Now it's time to move on to practice. To better understand how to write your own trading robot, you can learn the codes of existing similar programs.
MQL4 seems to be a complicated language only at first glance. In fact, if you spend some time studying it, you will soon be able to create your own automated systems that will help you daily in trading on financial markets.
Share
Related articles
- Previous article: Merry Christmas and Happy New Year!
- Next article: How to create own trading strategy and learn to predict the Forex market?